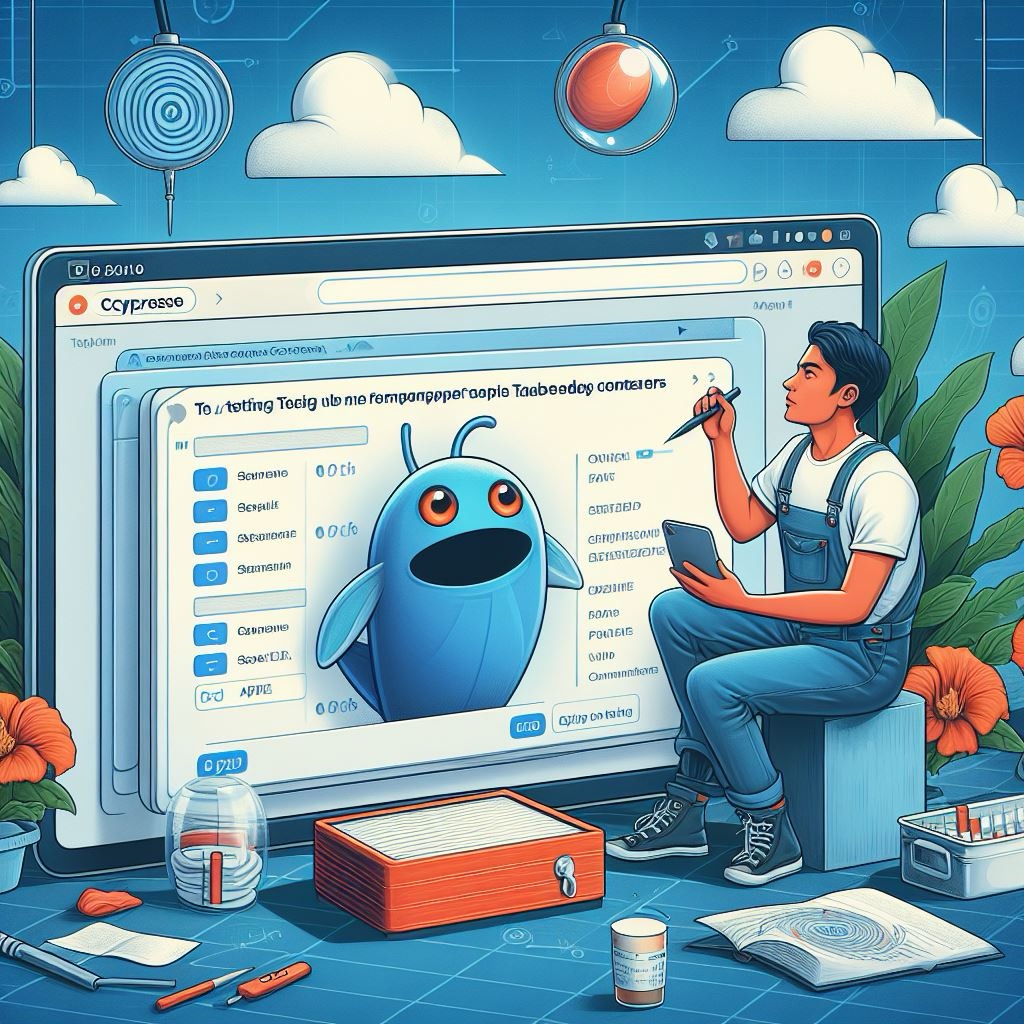
Introduction
Oracle APEX applications often use tabbed containers to organize information and functionality. While convenient for users, testing these dynamic elements can be tricky. Traditional automation frameworks often require complex selectors and workarounds. This is where Cypress, a modern JavaScript-based tool, shines. Cypress simplifies APEX testing with its intuitive API and ability to directly interact with DOM elements.
The Challenge: Identifying Tabs in Cypress
Cypress offers several methods for interacting with UI elements, but identifying tabs within containers presents a unique challenge. Basic selectors like cy.get()
might not be robust enough, especially when dealing with dynamically generated content or custom themes. Here are some common problems:
- Dynamic IDs or classes: Tab elements might have dynamically generated IDs or classes, making them difficult to locate consistently.
- Nested elements: Tabs might be nested within other elements, requiring complex selector chains.
- Visual attributes: Relying on visual attributes like text content or position can be brittle and prone to unexpected changes.
Cypress to the Rescue!
Fortunately, Cypress provides several methods to overcome these challenges and effectively test tabbed containers in your APEX application. Let’s explore some powerful techniques:
1. Using cy.contains()
for Tab Text:
This method locates elements based on their text content. It’s a reliable option when tab labels are unique and static.
JavaScriptcy.get('.apex_tab_header').contains('My Awesome Tab').click();
Use code with caution. Learn more
2. Leveraging Custom Attributes:
APEX allows adding custom attributes to DOM elements. You can leverage these attributes in your Cypress selectors for precise targeting.
JavaScriptcy.get('[data-tab-name="Important Data"]').click();
Use code with caution. Learn more
3. Utilizing cy.get()
with Unique Identifiers:
If your tabs have unique IDs or classes, you can use cy.get()
directly. Ensure these identifiers are stable and not dynamically generated.
JavaScriptcy.get('#myUniqueTabID').click();
Use code with caution. Learn more
4. Navigating by Index:
Sometimes, the order of tabs is important in your test scenario. Cypress allows navigating tabs by their index within the container.
JavaScriptcy.get('.apex_tab_header').eq(1).click(); // Clicks the second tab
Use code with caution. Learn more
5. Employing Utility Methods:
For improved code reusability and maintainability, consider creating custom utility methods that encapsulate the logic for interacting with tabs. This helps keep your test scripts clean and organized.
Example Utility Method:
JavaScriptfunction clickTabByName(tabName) { cy.get('.apex_tab_header').contains(tabName).click(); } // Usage in your test script clickTabByName('Settings');
Use code with caution. Learn more
Beyond Clicking: Validating Tab Content
Testing goes beyond simply clicking tabs. You’ll need to verify the content displayed after switching. Cypress offers powerful assertion methods to achieve this.
cy.contains()
: Verify if specific text is present within the tab content.cy.get()
with element-specific assertions: Check for the existence of specific UI elements or their attributes within the active tab.- Visual testing tools: Integrate visual testing libraries like Applitools Eyes to capture and compare screenshots of the tab content for visual consistency.
Remember:
- Test different scenarios like clicking disabled tabs, handling unexpected content, and verifying error messages.
- Leverage Cypress’s built-in debugging tools to troubleshoot any issues in your test scripts.
- Document your test cases clearly and maintain them regularly as your application evolves.
By employing these techniques and best practices, you can effectively test tabbed containers in your APEX application with Cypress, ensuring a smooth and reliable user experience